下圖是最終我們希望得到的結果:當表格中的 text 2 欄位文字過長時,可以被自動截斷,並加上刪節號。不過在實作的過程中,有遇到一些問題。以下是遇到的問題與解決方式:

首先,我們先做一個表格,外觀與程式碼如下:

<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> table { width: 100%; border: 1px solid #f00; border-collapse: collapse; } th, td { border: 1px solid #f00; } </style> </head> <body> <table> <thead> <tr> <th>head 1</th> <th>head 2</th> </tr> </thead> <tbody> <tr> <td>text 1</td> <td>text 2</td> </tr> <tr> <td>text 3</td> <td>text 4</td> </tr> </tbody> </table> </body> </html>
接著,我們將 text 2 這欄放入大量的文字,並在td第二欄使用css: overflow: hidden; white-space: nowrap; text-overflow: ellipsis; 期待能在文字超出範圍時,會顯示刪節號。程式碼如下:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> table { width: 100%; border: 1px solid #f00; border-collapse: collapse; } th, td { border: 1px solid #f00; } td:nth-child(2) { overflow: hidden; white-space: nowrap; text-overflow: ellipsis; } </style> </head> <body> <table> <thead> <tr> <th>head 1</th> <th>head 2</th> </tr> </thead> <tbody> <tr> <td>text 1</td> <td>text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2</td> </tr> <tr> <td>text 3</td> <td>text 4</td> </tr> </tbody> </table> </body> </html>
結果,我們卻發現,文字不但沒有被加上刪節號,反而表格還被撐大了,還使瀏覽器出現了水平卷軸(右方的粗黑線是瀏覽器的邊界)。
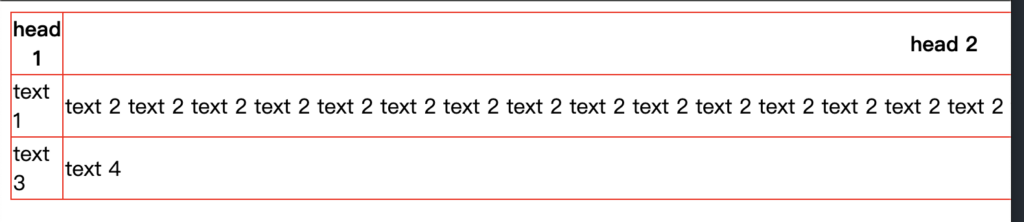
即使我們將 text 2 這欄的 td 設定一個大小,例如:200px,又或者把 text 2 的內容包在一個 div 中,且在此 div 設定刪節號的 css,結果也都是一樣無法正常顯示。
最後找到一個非常簡單的解決方式,只需將該 table 的 css 加上 table-layout: fixed,一切都解決了。
table-layout 這個屬性所掌管的,其實就是表格總寬度的計算,共有兩種值可以使用,一個是 automatic(以每欄內容寬度計算,此為預設值),另一個是 fixed(以 table 設定的 width 屬性為準)
我們試試看,如果不設定此屬性,或將其設定為預設值 automatic,即使我們將 table 設定 width: 100% 時,在文字不超出的情況下,雖然正常顯示,但一旦有一張超大的圖或者無法換行的文字等等,表格總寬度就會被撐開。但當我們設定為 fixed 時,雖然超出範圍的東西還是超出,但明顯地,表格總寬度被限制了,欄位寬度也不再是浮動的,也就代表著內部的 overflow 可以被正常運作(overflow 無法運作的情形,通常都是因為父層的寬度是浮動的,導致無法得知需要被限制的寬度為多少)。
使用 table-layout: fixed 的畫面與程式碼如下:

<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> table { width: 100%; border: 1px solid #f00; border-collapse: collapse; table-layout: fixed; } th, td { border: 1px solid #f00; } td:nth-child(2) { overflow: hidden; white-space: nowrap; text-overflow: ellipsis; } </style> </head> <body> <table> <thead> <tr> <th>head 1</th> <th>head 2</th> </tr> </thead> <tbody> <tr> <td>text 1</td> <td>text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2</td> </tr> <tr> <td>text 3</td> <td>text 4</td> </tr> </tbody> </table> </body> </html>
這樣就成功一半了!為什麼說是一半呢?由上圖我們會發現,一但加上了 table-layout: fixed 每欄的寬度會被固定成一樣大。但通常我們會需要特別設定一些欄位的寬度,這時,我們只要針對該欄的th與td都設定好寬度即可,可以是任何單位。如果只設定th, td其中一個會不起作用呦。設定完後的結果與程式碼如下:

<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> table { width: 100%; border: 1px solid #f00; border-collapse: collapse; table-layout: fixed; } th, td { border: 1px solid #f00; } th:nth-child(1), td:nth-child(1) { width: 120px; /* 百分比或其他單位也可以 width: 20%; */ } th:nth-child(2), td:nth-child(2) { /* 百分比或其他單位也可以 width: 80%; */ overflow: hidden; white-space: nowrap; text-overflow: ellipsis; } </style> </head> <body> <table> <thead> <tr> <th>head 1</th> <th>head 2</th> </tr> </thead> <tbody> <tr> <td>text 1</td> <td>text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2 text 2</td> </tr> <tr> <td>text 3</td> <td>text 4</td> </tr> </tbody> </table> </body> </html>
在〈[css筆記] 在表格中使用刪節號 css text-overflow: ellipsis〉中有 1 則留言